Base64 Encoding for Media in Flutter
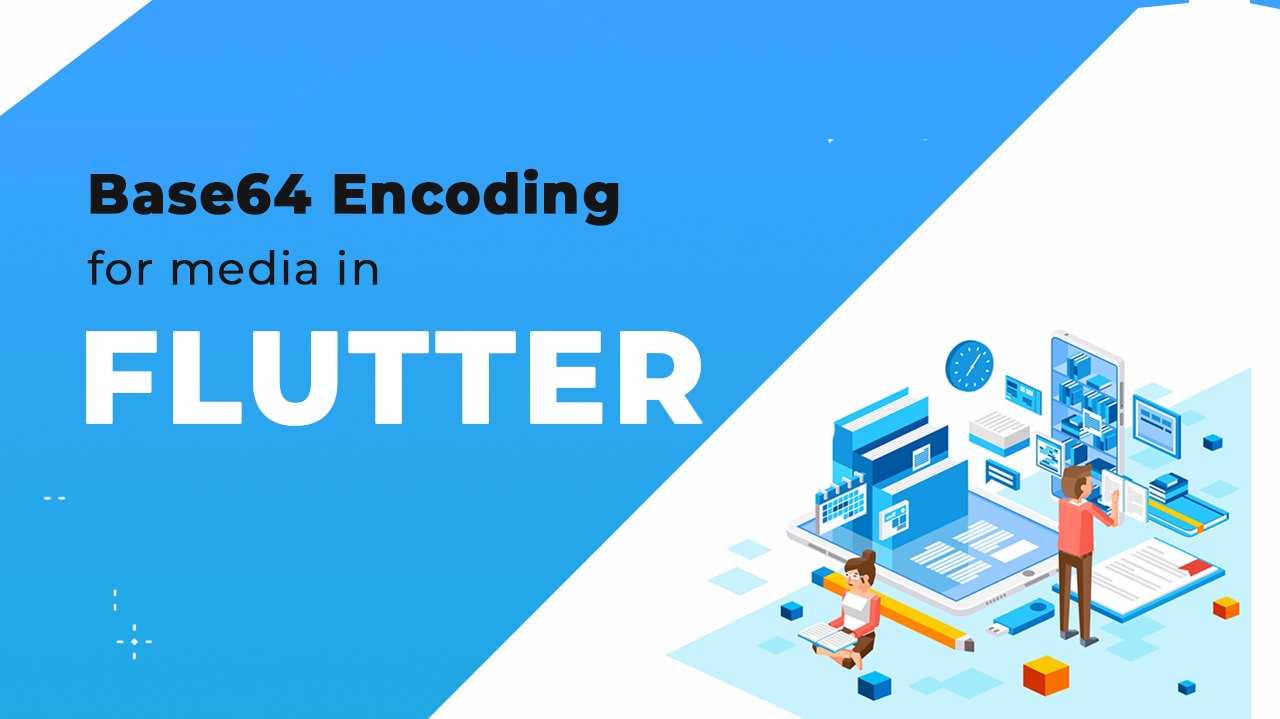
In mobile application development, we sometimes come across the feature wherein we need to encode a media file such as image, video, etc. Base64 is one of the available options for us to encode the object like strings and media. An encoded object will be then available as a Base64 string and ready-to-go in our API request parameters. It should be very straightforward to do so for any platform. Like in Flutter mobile development, we can pick a media using the FilePicker package which is available as a File object. This File object can encode as Base64 string easily:
FilePicker.getFile(type: FileType.image).then((file) {
final bytes = file.readAsBytesSync();
final base64Str = base64Encode(bytes);
});
So the single line to base64 encode any file will be:
String base64String = base64Encode(file.readAsBytesSync());
Note: The File object is only available for mobile and not for flutter web, as its from dart:io package which is not exposed to flutter web.
Well, encoding the media to base64 might be useful in a case when we want to upload it on server by sending it in a parameters of API request. What if we want to read media from url as base64 string directly?
Getting base64 string from a media URL
My thought was to download the media first, then convert it as a File object, then convert it to base64 string. But this will be limited to Flutter mobile only and will not work for Flutter web. So let us go ahead to look for one unique solution that will work for both mobile and web, and here see what I found it is easy using the http package.
static Future<String> networkImageToBase64(String mediaUrlString) async {
http.Response response = await http.get(mediaUrl);
final bytes = response?.bodyBytes;
return (bytes != null ? base64Encode(bytes) : null);
}
We just pass a media URL to this function and we will get the base64 encode string for that media:
final imgBase64Str = await networkImageToBase64("YOUR_MEDIA_URL_STRING");
This worked perfectly for both mobile and web.